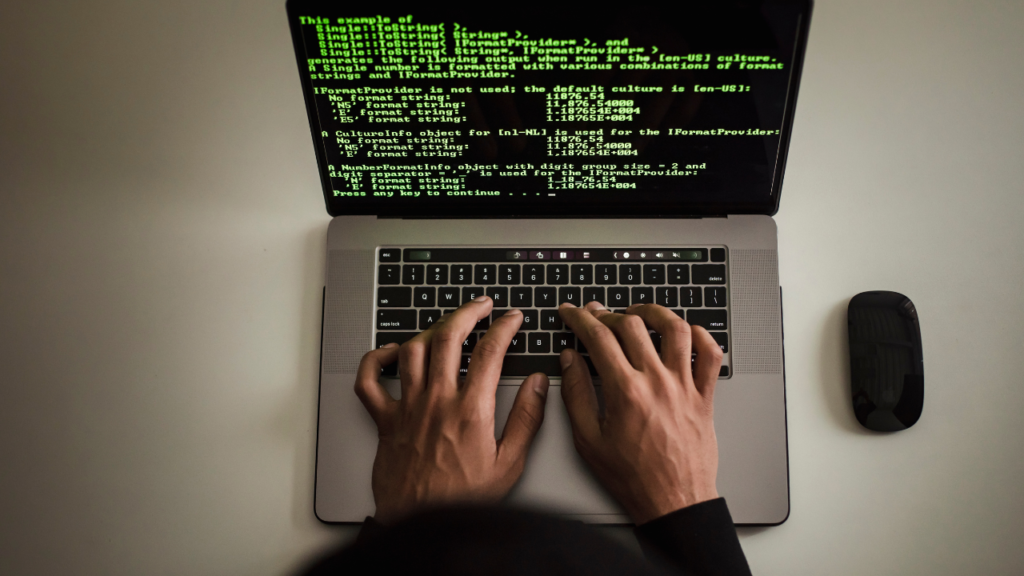
General programming interview questions #
- Describe the process of writing a piece of code from requirements to delivery.
- Which tools have you used to test your code quality?
- How can you debug a program while it’s being used?
- What’s your favorite programming language and why? What features (if any) would you like to add to this language?
- Where do you place most of your focus when reviewing somebody else’s code?
- How do you stay up-to-date with the latest technology developments?
For junior programmers #
- What programming languages are you most familiar with?
- What JavaScript engines do you know of?
- What is the difference between an abstract class and an interface and when would you use one over the other?
- Describe the troubleshooting process you’d follow for a crashing program.
- How can you debug a program while it’s being used?
- Describe the programming processes at work from the time you type in a website’s URL to when it finishes loading on your screen.
- Can you name some limitations of a web environment vs. a Windows environment?
- Are you familiar with cloud systems? What are their pros and cons?
- What is your field of expertise and what would you like to learn more about?
For senior programmers #
- Have you implemented significant improvements to an IT infrastructure? What were they?
- We want to install a new software system. What would be your research method and what steps would you follow before deployment?
- What’s the most effective way to gather user and system requirements?
- Describe a situation where you had to explain technical details to a non-technical audience. How did you modify your presentation?
- What guidance would you provide a new team member?
- What’s the most challenging project you’ve managed so far? What was your role and how did you manage your work to deliver on deadlines?
Java coding interview questions #
Computer Science Questions #
- How do you know if a linked list has a cycle in it? As in, one of the nodes in the linked list points to a previous node in the list.
- Describe the characteristics of an ACID database system.
- Is Java a statically or dynamically typed language?
- What do we mean by polymorphism, inheritance and encapsulation?
- Do arguments in Java get passed by reference or by value?
- What is the difference between an abstract class and an interface and when would you use one over the other?
- Why use an object Factory and how would you implement the Singleton pattern?
- What is the difference between “==” and equals(…) method? What is the difference between shallow comparison and deep comparison of objects?
- How is Java EE related to Java SE?
- How are Runtime exceptions different from Checked exceptions?
- What is the difference between HashMap, ConcurrentHashMap and a Map returned by Collections.synchronizedMap?
- Servlets 3.0 introduced async support. Describe a use case for it.
- Why do you think lambda expressions are considered such a big thing in Java 8?
- Given this code fragment:
class Engine { }
public class App {
public static void main(String[] args) {
Engine e = new Engine();
Engine e1 = e; e = null;
}
} - Which statement is true about this code?
- It creates an object and the object is eligible for garbage collection.
- It creates an object and the object is not eligible for garbage collection.
- It creates two objects: e and e1. The e-object is eligible for garbage collection.
- It creates two objects and both objects are ineligible for garbage collection.
- Is this possible in Java?
A extends B, C
Ruby coding interview questions #
- What is the use of load and require in Ruby?
- Explain each of the following operators and how and when they should be used: ==, ===, eql?, equal?
- What is a module? Can you tell me the difference between classes and modules?
- What are some of your favorite gems?
Role-specific questions #
- Do arguments in Ruby get passed by reference or by value?
- Is Ruby a statically or dynamically typed language?
- What do you mean by polymorphism, inheritance and encapsulation?
- What tools do you use for linting, debugging and profiling?
- Describe the three levels of method access control for classes and modules.
- What do they imply about the method?
- What’s duck typing? Describe a use case.
- How is a block different from a Proc ?
- Ruby MRI uses a Global Interpreter Lock. Does that mean it doesn’t use real threads?
- What do we mean when we say that a lambda or block forms a closure?
- What’s the following program going to print?
x = 1
class MyClass
y = 2
def foo
z = 4
y ||= 0
puts z + y
end
define_method :bar do |x|
z = 5
y ||= 0
puts z + y + x
end end my_class = MyClass.new
my_class.foo
my_class.bar(10)
my_class.bar
Python coding interview questions #
- Why are functions considered first class objects in Python?
- Can you explain circular dependencies in Python and potential ways to avoid them?
- Give an example of filter and reduce over an iterable object.
- Can you explain the uses/advantage of a generator?
- Give an example of filter and reduce over an iterable object
- Implement the linux whereis command that locates the binary, source, and manual page files for a command.
- What are list and dict comprehensions?
- What do we mean when we say that a certain Lambda expression forms a closure?
- What is the difference between list and tuple?
- What will be the output of the following code?
list = ['a', 'b', 'c', 'd', 'e']
print list[10:] - What will be the output of the following code in each step?
class C:
dangerous = 2
c1 = C()
c2 = C()
print c1.dangerous
c1.dangerous = 3
print c1.dangerous
print c2.dangerous
del c1.dangerous
print c1.dangerous
C.dangerous = 3
print c2.dangerous
.NET coding interview questions #
- What is the WebSecurity class in .NET? What is its use?
- In .NET, attributes are a method of associating declarative information with C# code. Please describe the way they are used and a proper use case.
- Which is the best way to pass configuration variables to ASP.NET applications?
- Is it possible in .NET to extend a class (any class) with some extra methods? If yes, how can it be accomplished?
- What do you mean by polymorphism, inheritance and encapsulation?
- What is the difference between an abstract class and an interface and when would you use one over the other?
- What do we mean when we say that a certain Lambda expression forms a closure?
- Is it possible in .NET to extend a class (any class) with some extra methods? If yes, how can it be accomplished?
- What are multi-cast delegates?
- In .NET, attributes are a method of associating declarative information with C# code. Please describe the way they are used and a proper use case.
- What is the WebSecurity class in .NET? What is its use?
- Which is the best way to pass configuration variables to ASP.NET applications?
- Can the web.config file contain user defined sections? How are they declared and accessed by code?
- Please describe the process of deploying a Web Application using Web Deploy. How is the web.config file altered automatically to contain the proper configuration for the deployment environment?
PHP coding interview questions #
- If you need to generate random numbers in PHP, what method would you follow?
- What’s the difference between the include() and require() functions?
- Explain how you develop and integrate plugins for PHP frameworks, like Laravel and Yii.
- How can you get web browser details using PHP?
Behavioral questions #
- What’s a fun project that you’ve worked on recently?
- If you could attend any tech seminar, which one would you choose and why?
- How do you ensure you discover all current programming trends?
- Describe a situation where you collaborated with developers and engineers to complete a project. What was your contribution to the team?
HTML/CSS coding interview questions #
- Can you describe the difference between inline elements and block elements?
- Why is it generally a good idea to position CSS <link>s between <head></head> and JS <script>s just before </body>? Do you know any exceptions?
- Can you describe how padding and margin differ?
- How is responsive design different from adaptive design?
How to evaluate candidates’ answers #
Since knowing HTML is basically a hard skill, there’s a definite red flag in candidate answers: not knowing the basics. Candidates should feel fairly comfortable answering knowledge questions (such as “what does this tag do?”) and be able to formulate a logical answer when presented with an HTML exercise.
For senior roles, you can also look at whether candidates seem comfortable making high-level decisions (for example, how they would improve your website’s layout). Also, keep an eye out for candidates with wide experience in using HTML in tandem with CSS and JavaScript.
JQuery interview questions #
- How would you delete cookies in JQuery?
- What are the basic JQuery selectors and how do you use them?
- Is there a difference between jquery.size() and jquery.length?
- What are the advantages of JQuery?
JavaScript interview questions #
- Which are the different types of variables?
- What is the pop()method in JavaScript?
- What’s a “closure” in JavaScript?
- Can you explain how you would clone an object?